Email Template
An email template is a predefined model used to generate emails in a standardized way. Instead of manually composing an emailđź“§ each time it is to be sent, a template provides a reusable framework that includes text, formatting, and dynamic elements (such as the recipient's name, dates or specific information). These dynamic elements are often automatically filled in by variables when the email is sent.
Ceate An Email Template
In Activator Admin, the stored function is located in the menu container as shown in (1) in the image below (this scenario is the same for other storedfunction components once their menu has been localized).
✋NB: Make sure you are in the System Component main module.
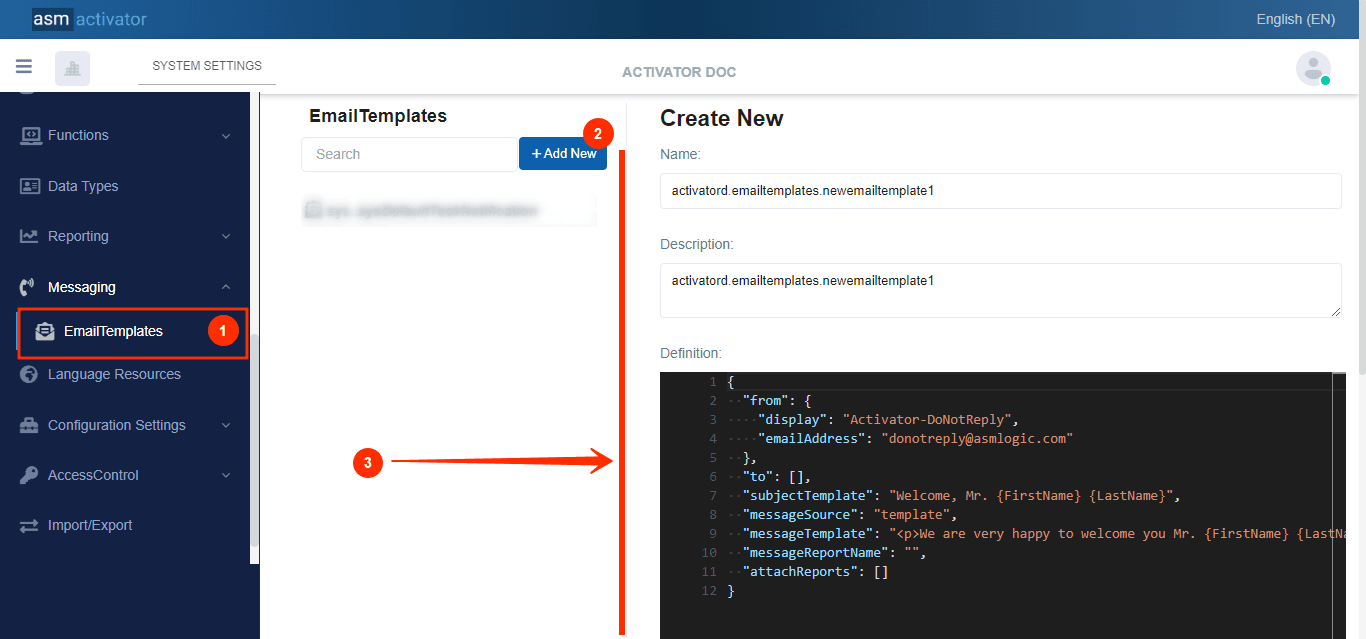
After initiating the creation of the Email template, by clicking on the +Add new button (illustrated at (2) on the image above), you’ll observe a form (illustrated at (3) on the image above) appearing on the right side with some fields.
🔬After filling in the name and description fields, let's focus on the Definition and Meta Data fields.
The Meta Data field gathers and provides additional information for the component, please refer to Meta Data for the complete list of additional information related to this component
As for the Definition field, let's take a closer look at its Json content in the next point.
Definition Content
Here's the code defined to create an email template.
{
"from": {
"display": "Activator-DoNotReply",
"emailAddress": "donotreply@asmlogic.com"
},
"to": [],
"subjectTemplate": "Welcome, Mr. {FirstName} {LastName}",
"messageSource": "template", // or "report"
"messageTemplate": "<p>We are very happy to welcome you Mr. {FirstName} {LastName}</p>",
"messageReportName": "",
"attachReports": []
}
Description
PROPERTY | REQUIRED | DEFAULLT VALUE | DESCRIPTION |
---|---|---|---|
from | Yes |
| Contains sender information. With two properties:
|
to | Yes | [] | Recipient list. Can contain multiple email addresses, especially with display and emailAddress properties. |
subjectTemplate | Yes | String | Email subject template with parameters such as {FirstName} , {LastName} for customization. |
mesageRessource | Yes | template | Defines the message source. Can be either template (uses a message model) or report (which is another component explained here). |
messageTemplate | Conditional | String | If messageSource is set to template then it will Contain the HTML template of the email body with parameters to customize the content. |
messageReportName | Conditional | "" | If messageSource is set to report then it contains the name of the report used to generate the email body. |
attachReports | No | "" | List of reports to attach to the email. Each item can represent a report to be included in the email. |
Parameter
The {FirstName}
and {LastName}
parameters in an email template are dynamic parameters used to personalize the content of the email according to the recipient. These parameters are automatically replaced by the actual values when the email is sent.
How to Add Attachments
To add a report from the attachReport property, you need to include the properties for each report you wish to add:
{
"reportName": "activatord.reports.reportDemo",
"format": "pdf",
"fileName": "catalog.pdf"
}
How To Execute An Email Template
To execute an email template, it is done exclusively in stored functions.
Below is the code that allows execution.
bool result = await context.Notifications.SendEmailFromTemplate(
"activatord.emailtemplates.emailTemplateName",
to,
null, //subject Fields params values
null, //message Fields params values
reportParameters,
fileAttachments
);
Here's a table describing the code parameters for executing an email template with their characteristics:
PROPERTY | Type | DESCRIPTION |
---|---|---|
activatord.emailtemplates.emailTemplateName | String | Name of the email template used to send the message. This name corresponds to an email template defined in the system |
to | List<string> or string | List of email recipients. Can be one or several emails (in a table or a list). |
null (subject fields) | string | Values to replace in email subject parameters (e.g. {FirstName} , {LastName} ). If no value to replace, can be null. |
null (message Fields) | string | Values to replace in message body parameters (e.g. {FirstName}, {LastName}). If no value to replace, can be null. |
reportParameters | object | Parameters used if the email is generated from a report. These are the parameters that will be used to run the report. |
fileAttachments | List<FileAttachment> | List of files to be attached to the email. Each item corresponds to an attached file. |
✋Note:
The
to
property specified in the code is added to any list of addresses already defined in the email template. This means that the recipients passed as a parameter will be added to those initially defined during the template creation, without replacing them.- Similarly, the
fileAttachments
specified in the code are added to the list of files defined in theattachReports
property of the email template. The attachments passed as a parameter will be added to those already present in the template, if any.This ensures that additional addresses and files are added to the existing information without overwriting what was preconfigured in the email template.
Examples
Our email subject line reads “Hi {firstName}
{lastName}
”.
Example of use with a report
In this case, it's essential to note that the messageSource
property defined in the email template must be set to report
. This indicates📍 that the message content will be generated from a report rather than a simple text template.
var to = new List<EmailAddressModel>(); //Defining the recipient list
var reportParameters = new List<EmailTemplateReportParameters>(); //Defining report parameters
var fileAttachments = new List<EmailMessageAttachment>(); //List of attachments
//a recipient is added:
to.Add(new EmailAddressModel
{
Display = "John Doe",
EmailAddress = "john.doe@example.com"
});
//We add a report parameter:
reportParameters.Add(new EmailTemplateReportParameters
{
ReportName = "activatord.reports.reportName",
Parameters = new List<ReportParameter>
{
new ReportParameter
{
Name = "Param Name",
Value = "Param Value"
}
}
});
//Setting field parameters for email subject :
var subjectFields = new {
firstName = "John",
lastName = "Doe",
};
//Send email via template :
bool result = await context.Notifications.SendEmailFromTemplate(
"activatord.emailtemplates.emailTemplateName",
to,
subjectFields,
null,
reportParameters,
fileAttachments
);
Example of use without report using an attachment
In this exampleđź’ˇ, the email content is based on a predefined text template, and not on a report. Therefore, the messageSource
field in the email template definition must be set to template
. This indicates that the email content will be generated from a text template (here, a simple HTML with parameters).
Let's consider that the content of our email is as follows:
======
<p>
Dear {ClientName}<br/>,
We confirm that your order number {OrderNumber} has been successfully processed. We will keep you informed of its status.<br/><br/>
Best regards,<br/>
ASM Activator
</p>
======
{ClientName}
and{OrderNumber}
are parameters which will be replaced by the values supplied when the template is run.
Code for use:
var to = new List<EmailAddressModel>();//Defining the recipient list
var reportParameters = new List<EmailTemplateReportParameters>();//null
var fileAttachments = new List<EmailMessageAttachment>(); //List of attachments
//Defining the recipient
to.Add(new EmailAddressModel
{
Display = "John Doe",
EmailAddress = "john.doe@example.com"
});
//Definition of message fields (parameters)
var messageFields = new {
ClientName = "John Doe",
OrderNumber = "123456789KL",
};
//Adding attachments
fileAttachments.Add(
new EmailMessageAttachment
{
FileName = "FileName.pdf",
FileBase64Content = base64EncodedContent //Base64 content of the file you want to attach
}
);
//Send email via template
bool result = await context.Notifications.SendEmailFromTemplate(
"activatord.emailtemplates.emailTemplateName",
to,
null,
messageFields,
reportParameters,//null
fileAttachments
);
Conclusion
Sendingđź“® emails via email templates in Activator allows for generating dynamic and personalized messages using HTML templates or reports. These mechanisms offer great flexibility in managing automated communications, while ensuring that static and dynamic information is combined without overwriting the preconfigured data in the template.
With a solid understanding of email templates and their dynamic capabilities, we can now explore how Email APIs Email APIs enhance the functionality and integration of email communication within Activator.